parent
41345d34b2
commit
40cd5e27ab
24
README.md
24
README.md
@ -15,7 +15,15 @@ Change the gui page title, favicon and app icons of your Home Assistant instance
|
||||
|
||||
There are some nice ones available [here](https://github.com/home-assistant/home-assistant-assets/tree/master/Alternates), and you can generate favicons from them using an online tool, such as [this one](https://realfavicongenerator.net/).
|
||||
|
||||
- Put your icons in e.g. `<your config dir>/www/favicons/`
|
||||
- Put your icons in e.g. `<your config dir>/www/favicons/`. Note that `<your config dir>/www/` translates to `/local/` for the Icon path.
|
||||
Note: If you created `<your config dir>/www/` you need to restart Home Assistant once before any icons will be found.
|
||||
|
||||
### About the icons
|
||||
`hass-favicon` will scan the specified directory and automatically apply icons when found based on their filename. There are three types of icons, and it's important that you get the filenames correct.
|
||||
|
||||
- `favicon.ico` - The icon which is displayed on the browser tab and in the bookmark menu
|
||||
- `favicon-apple-<anything>.png` - The icon which is displayed if you save your interface to the home screen of your iDevice.
|
||||
- `favicon-<size>x<size>.png` - Used by android devices. `<size>` indicates the icon size in pixels, e.g. `favicon-1024x1024.png`.
|
||||
|
||||
## Method 1/2 Integration
|
||||
|
||||
@ -23,13 +31,13 @@ Change the gui page title, favicon and app icons of your Home Assistant instance
|
||||
|
||||
- Add a "Favicon" integration
|
||||
|
||||
- Enter what you want the title of the Home Assistant interface page to be, and the URL of the icons you wish to change. E.g. `favicon URL: /local/favicons/favicon.ico`, `iOS icon URL: /local/favicons/180x180.png`.
|
||||
- Enter your wanted title and the path to your icons. E.g. `Icon path: /local/favicons/`
|
||||
|
||||
- Press submit
|
||||
|
||||
- Refresh the page
|
||||
- Refresh the page. Make sure to clear the cacke of your browser to get the new icons.
|
||||
|
||||
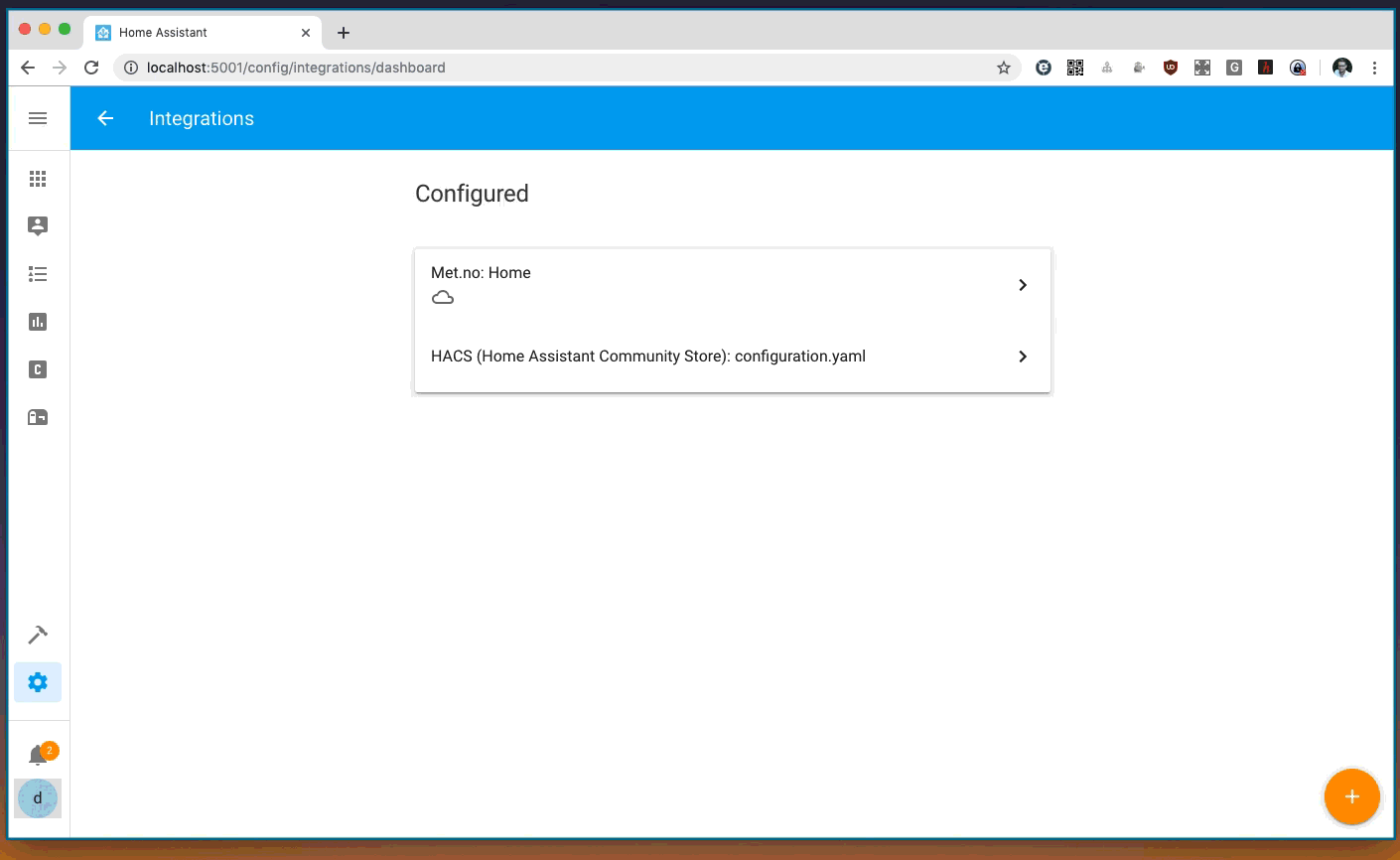
|
||||
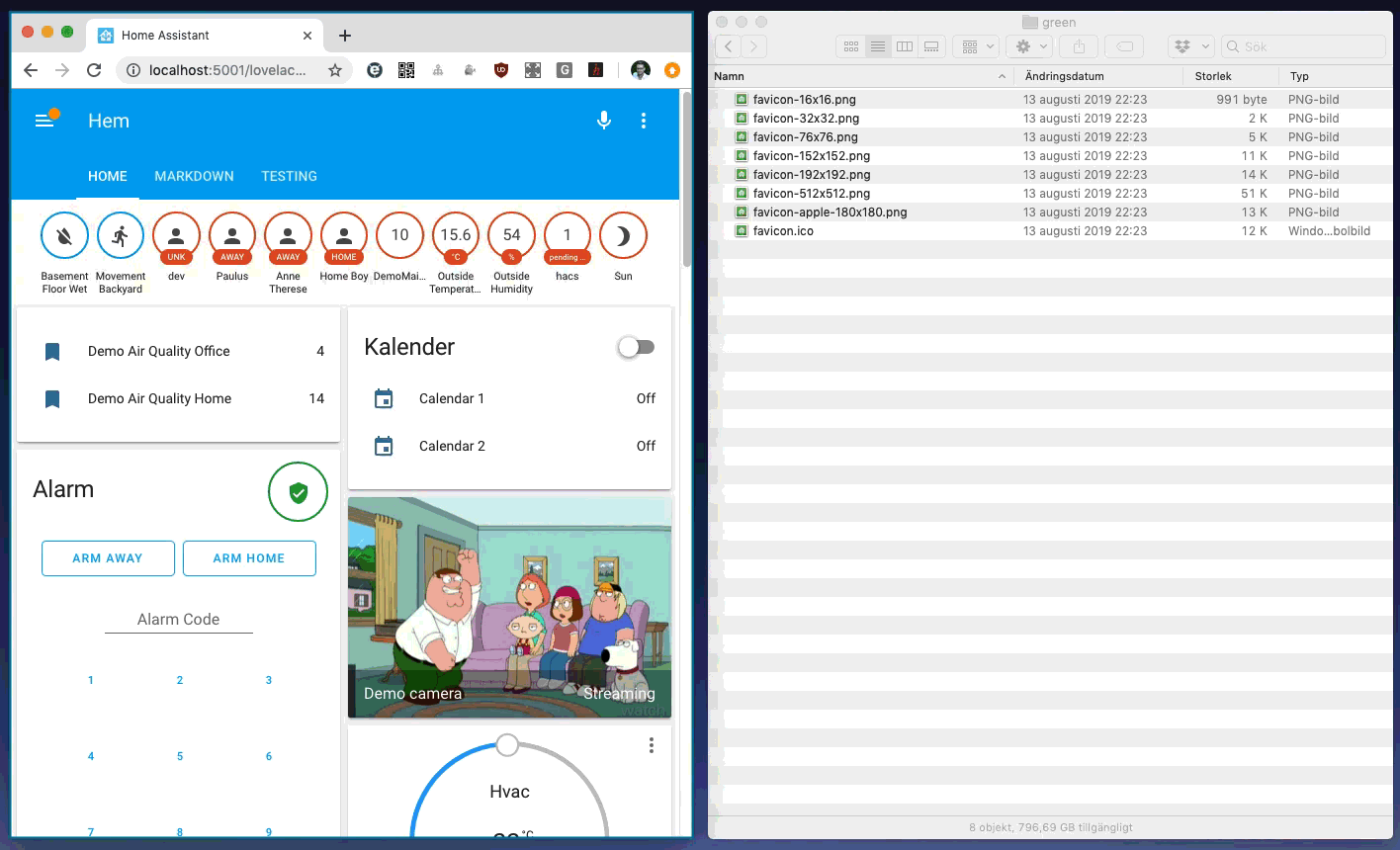
|
||||
|
||||
## Method 2/2 YAML configuration
|
||||
|
||||
@ -38,8 +46,7 @@ Change the gui page title, favicon and app icons of your Home Assistant instance
|
||||
```yaml
|
||||
favicon:
|
||||
title: My Home
|
||||
favicon: /local/favicons/favicon.ico
|
||||
apple: /local/favicons/apple-touch-icon-180x180.png
|
||||
icon_path: /local/favicons/
|
||||
```
|
||||
|
||||
- Restart Home Assistant
|
||||
@ -50,10 +57,7 @@ favicon:
|
||||
|
||||
- `title` - The title to display at the top of the window or browser tab.
|
||||
|
||||
- `favicon` - an .ico file which is displayed in your browser tab or bookmark menu.
|
||||
|
||||
- `apple` - a 180 x 180 px image that will be displayed on your iDevice home screen if you save the link there
|
||||
|
||||
- `icon_path:` - The path (frontend path) of the directory containing your icons.
|
||||
|
||||
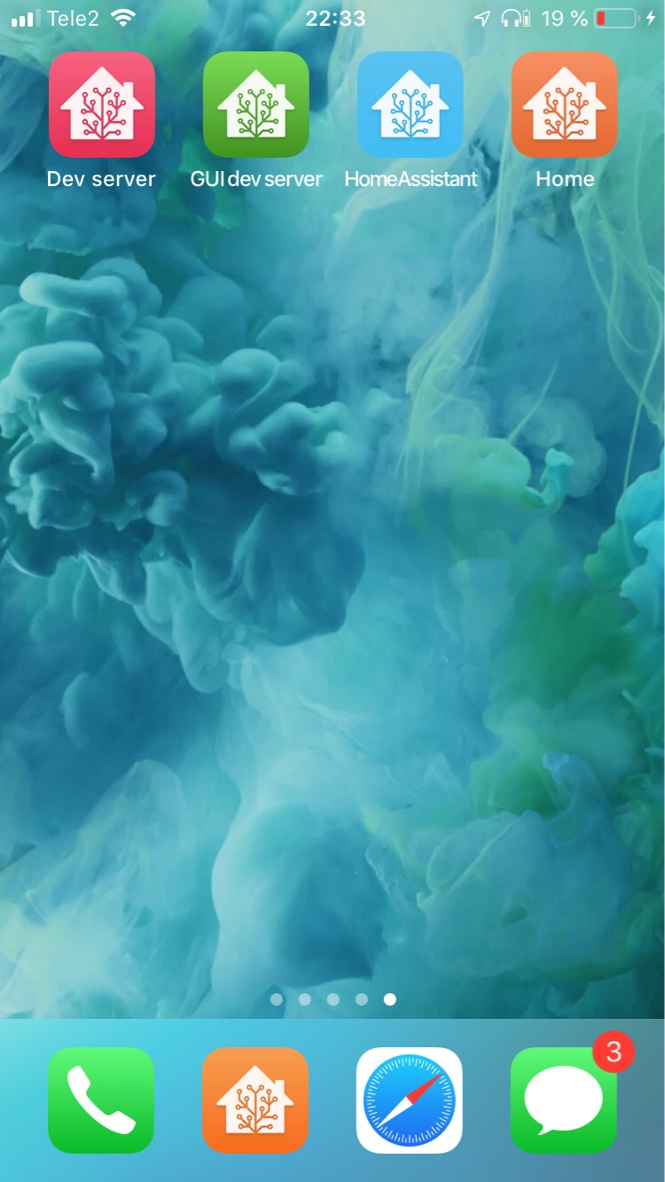
|
||||
|
||||
|
@ -4,11 +4,10 @@
|
||||
"step": {
|
||||
"config": {
|
||||
"title": "Favicon changer",
|
||||
"description": "Set the page title, favicon and/or iOS icons",
|
||||
"description": "Set the page title, and path to icons.",
|
||||
"data": {
|
||||
"title": "Page title",
|
||||
"favicon": "favicon URL",
|
||||
"apple": "iOS icon URL"
|
||||
"icon_path": "Icon path"
|
||||
}
|
||||
}
|
||||
},
|
||||
@ -21,8 +20,7 @@
|
||||
"init": {
|
||||
"data": {
|
||||
"title": "Page title",
|
||||
"favicon": "favicon URL",
|
||||
"apple": "iOS icon URL"
|
||||
"icon_path": "Icon path"
|
||||
}
|
||||
}
|
||||
}
|
||||
|
@ -1,4 +1,6 @@
|
||||
import logging
|
||||
import os
|
||||
import re
|
||||
from collections import defaultdict
|
||||
|
||||
import homeassistant.components.frontend
|
||||
@ -8,16 +10,28 @@ _LOGGER = logging.getLogger(__name__)
|
||||
|
||||
DOMAIN = "favicon"
|
||||
|
||||
RE_APPLE = "^favicon-apple-"
|
||||
RE_ICON = "^favicon-(\d+x\d+)\..+"
|
||||
|
||||
CONFIG_TITLE = "title"
|
||||
CONFIG_ICON_PATH = "icon_path"
|
||||
|
||||
async def async_setup(hass, config):
|
||||
if not hass.data.get(DOMAIN):
|
||||
hass.data[DOMAIN] = defaultdict(int)
|
||||
|
||||
if not hass.data[DOMAIN].get("get_template"):
|
||||
hass.data[DOMAIN]["get_template"] = homeassistant.components.frontend.IndexView.get_template
|
||||
if not hass.data[DOMAIN].get("manifest_icons"):
|
||||
hass.data[DOMAIN]["manifest_icons"] = homeassistant.components.frontend.MANIFEST_JSON["icons"].copy()
|
||||
|
||||
conf = config.get(DOMAIN)
|
||||
if not conf:
|
||||
return True
|
||||
if CONFIG_ICON_PATH in hass.data[DOMAIN]:
|
||||
del hass.data[DOMAIN][CONFIG_ICON_PATH]
|
||||
if CONFIG_TITLE in hass.data[DOMAIN]:
|
||||
del hass.data[DOMAIN][CONFIG_TITLE]
|
||||
hass.data[DOMAIN].update(conf)
|
||||
return apply_hooks(hass)
|
||||
|
||||
@ -31,34 +45,90 @@ async def async_remove_entry(hass, config_entry):
|
||||
|
||||
async def _update_listener(hass, config_entry):
|
||||
conf = config_entry.options
|
||||
_LOGGER.error(conf)
|
||||
if CONFIG_ICON_PATH in hass.data[DOMAIN]:
|
||||
del hass.data[DOMAIN][CONFIG_ICON_PATH]
|
||||
if CONFIG_TITLE in hass.data[DOMAIN]:
|
||||
del hass.data[DOMAIN][CONFIG_TITLE]
|
||||
_LOGGER.error(hass.data[DOMAIN])
|
||||
hass.data[DOMAIN].update(conf)
|
||||
_LOGGER.error(hass.data[DOMAIN])
|
||||
return apply_hooks(hass)
|
||||
|
||||
|
||||
def find_icons(hass, path):
|
||||
|
||||
icons = {}
|
||||
manifest = []
|
||||
if not path or not path.startswith("/local/"):
|
||||
return icons
|
||||
|
||||
localpath = "www" + path[len("/local"):]
|
||||
localpath = hass.config.path(localpath)
|
||||
_LOGGER.info("Looking for icons in: %s", localpath)
|
||||
for fn in os.listdir(localpath):
|
||||
if fn == "favicon.ico":
|
||||
icons["favicon"] = os.path.join(path, fn)
|
||||
_LOGGER.info("Found favicon: %s", os.path.join(path, fn))
|
||||
apple = re.search(RE_APPLE, fn)
|
||||
if apple:
|
||||
icons["apple"] = os.path.join(path, fn)
|
||||
_LOGGER.info("Found apple icon: %s", os.path.join(path, fn))
|
||||
icon = re.search(RE_ICON, fn)
|
||||
if icon:
|
||||
manifest.append({
|
||||
"src": os.path.join(path, fn),
|
||||
"sizes": icon.group(1),
|
||||
"type": "image/png",
|
||||
})
|
||||
_LOGGER.info("Found icon: %s", os.path.join(path, fn))
|
||||
|
||||
if manifest:
|
||||
icons["manifest"] = manifest
|
||||
return icons
|
||||
|
||||
def apply_hooks(hass):
|
||||
data = hass.data[DOMAIN]
|
||||
data = hass.data.get(DOMAIN, {})
|
||||
icons = find_icons(hass, data.get(CONFIG_ICON_PATH, None))
|
||||
title = data.get(CONFIG_TITLE, None)
|
||||
|
||||
def _get_template(self):
|
||||
tpl = data["get_template"](self)
|
||||
render = tpl.render
|
||||
|
||||
def new_render(*args, **kwargs):
|
||||
text = render(*args, **kwargs)
|
||||
if data["favicon"]:
|
||||
text = text.replace("/static/icons/favicon.ico", data["favicon"])
|
||||
if data["apple"]:
|
||||
text = text.replace("/static/icons/favicon-apple-180x180.png", data["apple"])
|
||||
if data["title"]:
|
||||
text = text.replace("<title>Home Assistant</title>", f"<title>{data['title']}</title>")
|
||||
if "favicon" in icons:
|
||||
text = text.replace("/static/icons/favicon.ico", icons["favicon"])
|
||||
if "apple" in icons:
|
||||
text = text.replace("/static/icons/favicon-apple-180x180.png", icons["apple"])
|
||||
if title:
|
||||
text = text.replace("<title>Home Assistant</title>", f"<title>{title}</title>")
|
||||
return text
|
||||
|
||||
tpl.render = new_render
|
||||
return tpl
|
||||
|
||||
if title or "favicon" in icons or "apple" in icons:
|
||||
homeassistant.components.frontend.IndexView.get_template = _get_template
|
||||
|
||||
homeassistant.components.frontend.MANIFEST_JSON["icons"] = data["manifest_icons"].copy()
|
||||
if "manifest" in icons:
|
||||
homeassistant.components.frontend.MANIFEST_JSON["icons"] = icons["manifest"]
|
||||
|
||||
homeassistant.components.frontend.MANIFEST_JSON["name"] = "Home Assistant"
|
||||
homeassistant.components.frontend.MANIFEST_JSON["short_name"] = "Assistant"
|
||||
if title:
|
||||
homeassistant.components.frontend.MANIFEST_JSON["name"] = title
|
||||
homeassistant.components.frontend.MANIFEST_JSON["short_name"] = title
|
||||
|
||||
return True
|
||||
|
||||
def remove_hooks(hass):
|
||||
data = hass.data[DOMAIN]
|
||||
homeassistant.components.frontend.IndexView.get_template = data["get_template"]
|
||||
homeassistant.components.frontend.MANIFSET_JSON["icons"] = data["manifest_icons"].copy()
|
||||
homeassistant.components.frontend.MANIFEST_JSON["name"] = "Home Assistant"
|
||||
homeassistant.components.frontend.MANIFEST_JSON["short_name"] = "Assistant"
|
||||
return True
|
||||
|
||||
|
@ -1,8 +1,11 @@
|
||||
import logging
|
||||
import voluptuous as vol
|
||||
|
||||
from homeassistant import config_entries
|
||||
from homeassistant.core import callback
|
||||
|
||||
_LOGGER = logging.getLogger(__name__)
|
||||
|
||||
@config_entries.HANDLERS.register("favicon")
|
||||
class ExampleConfigFlow(config_entries.ConfigFlow):
|
||||
async def async_step_user(self, user_input=None):
|
||||
@ -20,8 +23,7 @@ class ExampleConfigFlow(config_entries.ConfigFlow):
|
||||
data_schema=vol.Schema(
|
||||
{
|
||||
vol.Optional('title'): str,
|
||||
vol.Optional('favicon'): str,
|
||||
vol.Optional('apple'): str,
|
||||
vol.Optional('icon_path'): str,
|
||||
}
|
||||
),
|
||||
)
|
||||
@ -38,17 +40,17 @@ class ExampleEditFlow(config_entries.OptionsFlow):
|
||||
|
||||
async def async_step_init(self, user_input=None):
|
||||
if user_input is not None:
|
||||
_LOGGER.error(user_input)
|
||||
return self.async_create_entry(title="", data=user_input)
|
||||
return self.async_show_form(
|
||||
step_id='init',
|
||||
data_schema=vol.Schema(
|
||||
{
|
||||
vol.Optional('title',
|
||||
description="test",
|
||||
default=self.config_entry.options.get("title", "")): str,
|
||||
vol.Optional('favicon',
|
||||
default=self.config_entry.options.get("favicon", "")): str,
|
||||
vol.Optional('apple',
|
||||
default=self.config_entry.options.get("apple", "")): str,
|
||||
vol.Optional('icon_path',
|
||||
default=self.config_entry.options.get("icon_path", "")): str,
|
||||
}
|
||||
),
|
||||
)
|
||||
|
8
info.md
8
info.md
@ -13,20 +13,22 @@ Change the favicon and app icons of your Home Assistant instance
|
||||
|
||||
There are some nice ones available [here](https://github.com/home-assistant/home-assistant-assets/tree/master/Alternates), and you can generate favicons from them using an online tool, such as [this one](https://realfavicongenerator.net/).
|
||||
|
||||
- Put your icons in e.g. `<your config dir>/www/favicons/`
|
||||
- Put your icons in e.g. `<your config dir>/www/favicons/`. Note that `<your config dir>/www/` translates to `/local/` for the Icon path.
|
||||
Note: If you created `<your config dir>/www/` you need to restart Home Assistant once before any icons will be found.
|
||||
|
||||
- Go to your Home Assistant configuration and to Integrations
|
||||
|
||||
- Add a "Favicon" integration
|
||||
|
||||
- Enter what you want the title of the Home Assistant interface page to be, and the URL of the icons you wish to change. E.g. `favicon URL: /local/favicons/favicon.ico`, `iOS icon URL: /local/favicons/180x180.png`.
|
||||
- Enter your wanted title and the path to your icons. E.g. `Icon path: /local/favicons/`
|
||||
|
||||
- Press submit
|
||||
|
||||
- Refresh the page
|
||||
|
||||
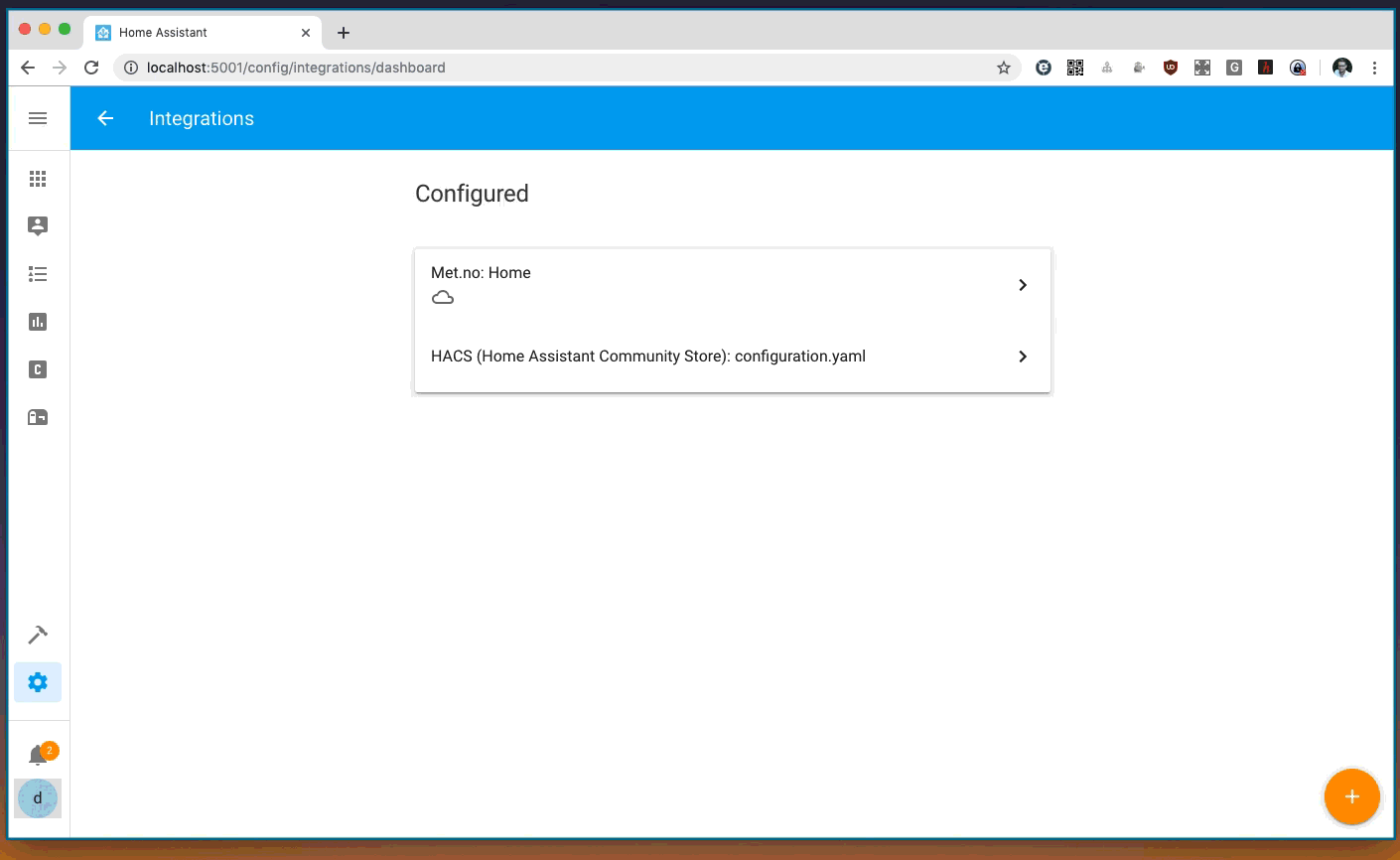
|
||||
See readme on github for more info about the icons.
|
||||
|
||||
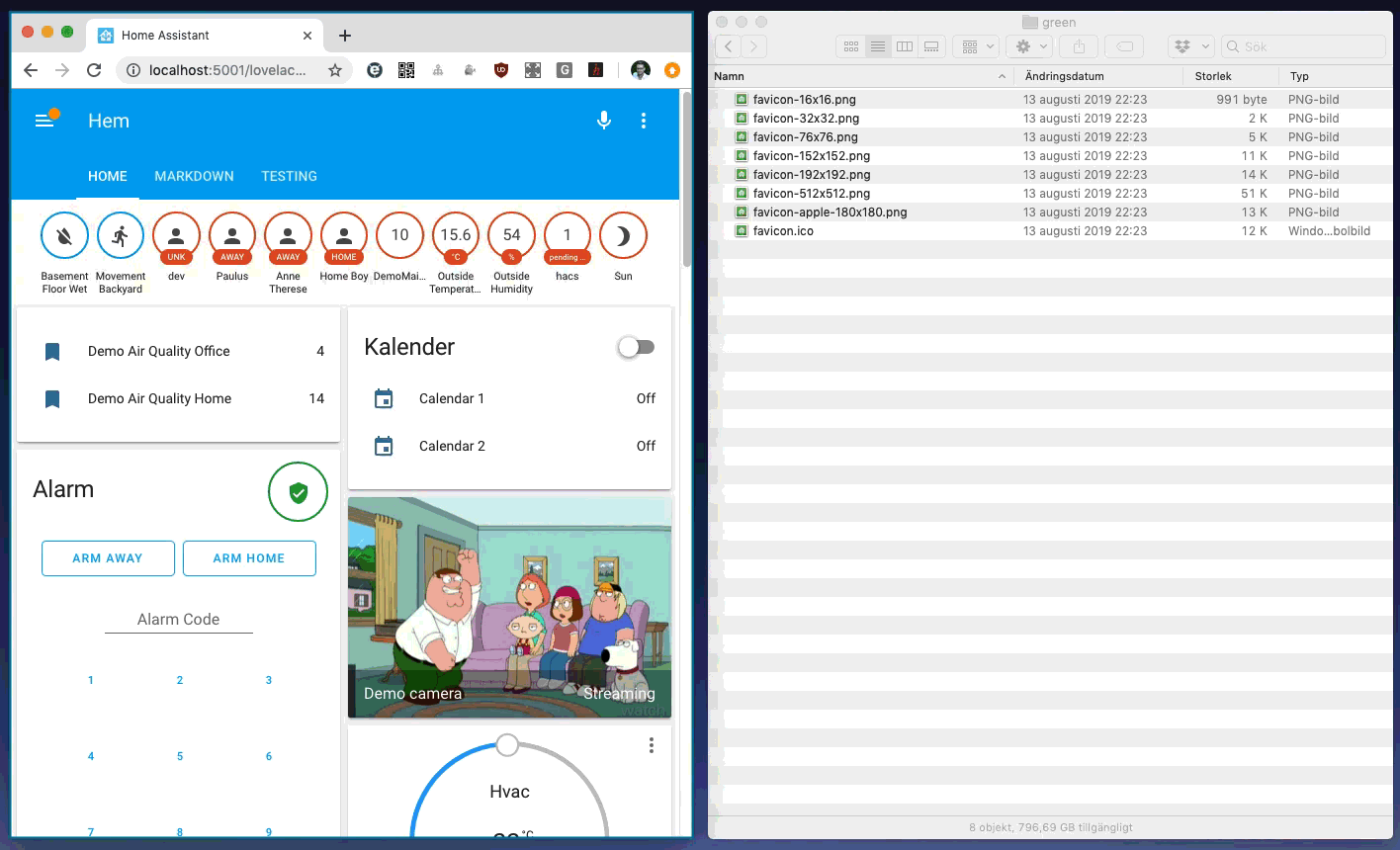
|
||||
|
||||
|
||||
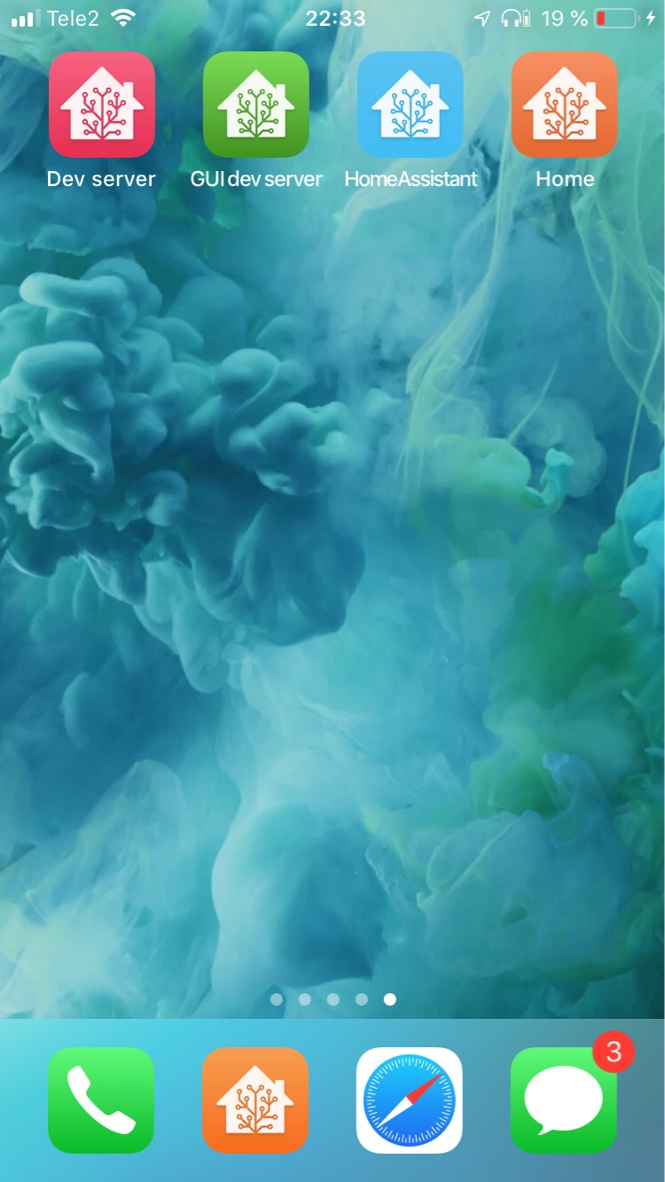
|
||||
|
Loading…
x
Reference in New Issue
Block a user