Initial release
This commit is contained in:
commit
1a68f90f23
40
README.md
Normal file
40
README.md
Normal file
@ -0,0 +1,40 @@
|
|||||||
|
folding-group-entity-row
|
||||||
|
========================
|
||||||
|
|
||||||
|
Display all entities in a group on a lovelace entities card - and fold it away when you don't want to see it.
|
||||||
|
|
||||||
|
---
|
||||||
|
|
||||||
|
```yaml
|
||||||
|
resources:
|
||||||
|
- url: /local/folding-group-entity-row.js
|
||||||
|
type: js
|
||||||
|
|
||||||
|
views:
|
||||||
|
- title: My view
|
||||||
|
cards:
|
||||||
|
- type: entities
|
||||||
|
entities:
|
||||||
|
- entity: group.my_group
|
||||||
|
type: custom:folding-group-entity-row
|
||||||
|
```
|
||||||
|
|
||||||
|
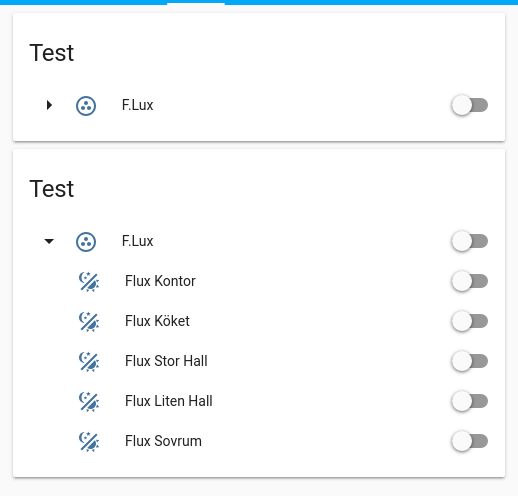
|
||||||
|
|
||||||
|
### Other options
|
||||||
|
|
||||||
|
- `config:` Config options to apply to each row in the group - same as for entity card
|
||||||
|
- `group_config:` Config options to apply to the group row - same as for entity card
|
||||||
|
|
||||||
|
Example:
|
||||||
|
```yaml
|
||||||
|
- entity: group.my_group
|
||||||
|
type: custom:folding-group-entity-row
|
||||||
|
config:
|
||||||
|
secondary_info: entity-id
|
||||||
|
group_config:
|
||||||
|
secondary_info: last-changed
|
||||||
|
type: custom:toggle-lock-entity-row
|
||||||
|
```
|
||||||
|
|
||||||
|
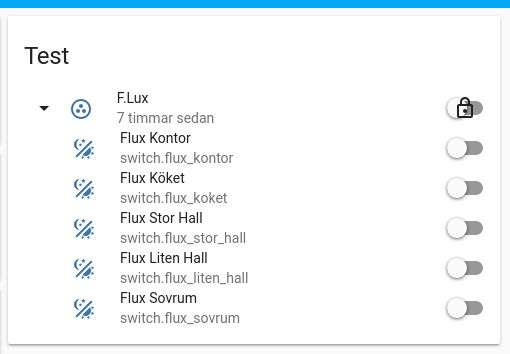
|
106
folding-group-entity-row.js
Normal file
106
folding-group-entity-row.js
Normal file
@ -0,0 +1,106 @@
|
|||||||
|
class FoldingGroupRow extends Polymer.Element {
|
||||||
|
|
||||||
|
static get template() {
|
||||||
|
return Polymer.html`
|
||||||
|
<style>
|
||||||
|
li, ul {
|
||||||
|
list-style: none;
|
||||||
|
}
|
||||||
|
ul {
|
||||||
|
transition: max-height 0.5s;
|
||||||
|
-webkit-transition: max-height 0.5s;
|
||||||
|
overflow: hidden;
|
||||||
|
padding: 0 0 0 40px;
|
||||||
|
margin: 0;
|
||||||
|
}
|
||||||
|
.closed > ul {
|
||||||
|
max-height: 0;
|
||||||
|
}
|
||||||
|
.open > ul {
|
||||||
|
max-height: 40px;
|
||||||
|
}
|
||||||
|
#head {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
}
|
||||||
|
.toggle {
|
||||||
|
width: 40px;
|
||||||
|
height: 40px;
|
||||||
|
align-items: center;
|
||||||
|
display: flex;
|
||||||
|
}
|
||||||
|
.toggle ha-icon {
|
||||||
|
flex: 0 0 40px;
|
||||||
|
}
|
||||||
|
</style>
|
||||||
|
<div id=head>
|
||||||
|
<div class=toggle on-click="doToggle">
|
||||||
|
<ha-icon icon="[[_icon]]"></ha-icon>
|
||||||
|
</div>
|
||||||
|
</div>
|
||||||
|
<li id="rows" class="closed">
|
||||||
|
</li>
|
||||||
|
`
|
||||||
|
}
|
||||||
|
|
||||||
|
update() {
|
||||||
|
this._icon = this.closed ? 'mdi:menu-right' : 'mdi:menu-down';
|
||||||
|
if(this.$)
|
||||||
|
this.$.rows.className = this.closed ? 'closed' : 'open';
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
doToggle(ev) {
|
||||||
|
this.closed = !this.closed;
|
||||||
|
this.update();
|
||||||
|
ev.stopPropagation();
|
||||||
|
}
|
||||||
|
|
||||||
|
ready() {
|
||||||
|
super.ready();
|
||||||
|
let conf = [Object.assign({entity: this._config.entity}, this._config.group_config || {})];
|
||||||
|
this._hass.states[this._config.entity].attributes.entity_id.forEach((e) => {
|
||||||
|
conf.push(Object.assign({entity: e}, this._config.config || {}));
|
||||||
|
});
|
||||||
|
this.dummy = document.createElement('hui-entities-card');
|
||||||
|
this.dummy.setConfig({entities: conf});
|
||||||
|
this.dummy.hass = this._hass;
|
||||||
|
this.appendChild(this.dummy);
|
||||||
|
|
||||||
|
let divs = this.dummy.shadowRoot.querySelector("ha-card").querySelector("#states");
|
||||||
|
let head = divs.firstChild.firstChild;
|
||||||
|
head.style.width = '100%';
|
||||||
|
this._addHeader(head);
|
||||||
|
while(divs.firstChild) {
|
||||||
|
this._addRow(divs.firstChild);
|
||||||
|
}
|
||||||
|
|
||||||
|
this.removeChild(this.dummy);
|
||||||
|
}
|
||||||
|
|
||||||
|
_addHeader(row)
|
||||||
|
{
|
||||||
|
this.$.head.appendChild(row);
|
||||||
|
}
|
||||||
|
_addRow(row)
|
||||||
|
{
|
||||||
|
let item = document.createElement('ul');
|
||||||
|
item.appendChild(row);
|
||||||
|
this.$.rows.appendChild(item);
|
||||||
|
}
|
||||||
|
|
||||||
|
setConfig(config) {
|
||||||
|
this._config = config;
|
||||||
|
this.closed = true;
|
||||||
|
this.update();
|
||||||
|
}
|
||||||
|
|
||||||
|
set hass(hass) {
|
||||||
|
this._hass = hass;
|
||||||
|
if(this.dummy)
|
||||||
|
this.dummy.hass = hass;
|
||||||
|
}
|
||||||
|
|
||||||
|
}
|
||||||
|
|
||||||
|
customElements.define('folding-group-entity-row', FoldingGroupRow);
|
Loading…
x
Reference in New Issue
Block a user