parent
9b36cf25bb
commit
a4edb45a14
19
README.md
19
README.md
@ -41,6 +41,7 @@ Currenly supported entity domains:
|
||||
- `min: <value>` - Set minimum value of slider
|
||||
- `max: <value>` - Set maximum value of slider
|
||||
- `step: <value>` - Set step size of slider
|
||||
- `attribute: <value>` - Select which attribute the slider should control
|
||||
|
||||
```yaml
|
||||
type: entities
|
||||
@ -76,5 +77,23 @@ entities:
|
||||
|
||||
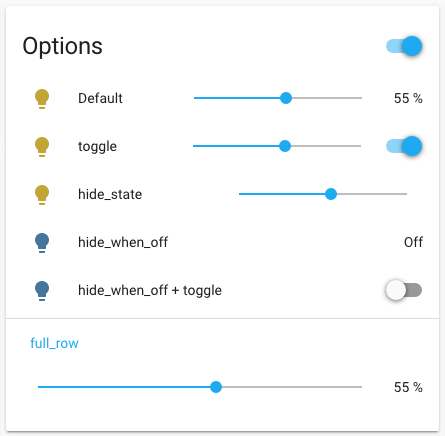
|
||||
|
||||
#### Attributes
|
||||
Currently, the following attribute settings are supported.
|
||||
|
||||
**For `light` domain:**
|
||||
|
||||
- `brightness` - default
|
||||
- `color_temp`
|
||||
- `hue`
|
||||
- `saturation`
|
||||
- `red`
|
||||
- `green`
|
||||
- `blue`
|
||||
|
||||
**For `cover` domain:**
|
||||
|
||||
- `position` - default
|
||||
- `tilt`
|
||||
|
||||
---
|
||||
<a href="https://www.buymeacoffee.com/uqD6KHCdJ" target="_blank"><img src="https://www.buymeacoffee.com/assets/img/custom_images/white_img.png" alt="Buy Me A Coffee" style="height: auto !important;width: auto !important;" ></a>
|
||||
|
File diff suppressed because one or more lines are too long
@ -2,25 +2,53 @@ import {Controller} from "./controller.js";
|
||||
|
||||
export class CoverController extends Controller {
|
||||
|
||||
get attribute() {
|
||||
return this._config.attribute || "position";
|
||||
}
|
||||
|
||||
get _value() {
|
||||
switch (this.attribute) {
|
||||
case "position":
|
||||
return this.stateObj.state === "open"
|
||||
? this.stateObj.attributes.current_position
|
||||
: 0;
|
||||
case "tilt":
|
||||
return this.stateObj.attributes.current_tilt_position;
|
||||
default:
|
||||
return 0;
|
||||
}
|
||||
}
|
||||
|
||||
set _value(value) {
|
||||
switch (this.attribute) {
|
||||
case "position":
|
||||
this._hass.callService("cover", "set_cover_position", {
|
||||
entity_id: this.stateObj.entity_id,
|
||||
position: value,
|
||||
});
|
||||
break;
|
||||
case "tilt":
|
||||
this._hass.callService("cover", "set_cover_tilt_position", {
|
||||
entity_id: this.stateObj.entity_id,
|
||||
tilt_position: value,
|
||||
});
|
||||
break;
|
||||
default:
|
||||
}
|
||||
|
||||
}
|
||||
|
||||
get string() {
|
||||
if (!this.hasSlider)
|
||||
return "";
|
||||
switch (this.attribute) {
|
||||
case "position":
|
||||
if (this.stateObj.state === "closed")
|
||||
return this._hass.localize("state.cover.closed");
|
||||
return `${this.value} %`
|
||||
case "tilt":
|
||||
return this.value;
|
||||
}
|
||||
}
|
||||
|
||||
get hasToggle() {
|
||||
@ -28,11 +56,19 @@ export class CoverController extends Controller {
|
||||
}
|
||||
|
||||
get hasSlider() {
|
||||
switch (this.attribute) {
|
||||
case "position":
|
||||
if ("current_position" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 4)) return true;
|
||||
case "tilt":
|
||||
if ("current_tilt_position" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 128)) return true;
|
||||
default:
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
get _step() {
|
||||
return 10;
|
||||
|
@ -2,18 +2,88 @@ import {Controller} from "./controller.js";
|
||||
|
||||
export class LightController extends Controller {
|
||||
|
||||
get attribute() {
|
||||
return this._config.attribute || "brightness";
|
||||
}
|
||||
|
||||
get _value() {
|
||||
return (this.stateObj.state === "on")
|
||||
? Math.ceil(this.stateObj.attributes.brightness*100.0/255)
|
||||
: 0;
|
||||
if (this.stateObj.state !== "on") return 0;
|
||||
switch (this.attribute) {
|
||||
case "color_temp":
|
||||
return Math.ceil(this.stateObj.attributes.color_temp);
|
||||
case "brightness":
|
||||
return Math.ceil(this.stateObj.attributes.brightness*100.0/255)
|
||||
case "red":
|
||||
return this.stateObj.attributes.rgb_color ? Math.ceil(this.stateObj.attributes.rgb_color[0]) : 0;
|
||||
case "green":
|
||||
return this.stateObj.attributes.rgb_color ? Math.ceil(this.stateObj.attributes.rgb_color[1]) : 0;
|
||||
case "blue":
|
||||
return this.stateObj.attributes.rgb_color ? Math.ceil(this.stateObj.attributes.rgb_color[2]) : 0;
|
||||
case "hue":
|
||||
return this.stateObj.attributes.hs_color ? Math.ceil(this.stateObj.attributes.hs_color[0]): 0;
|
||||
case "saturation":
|
||||
return this.stateObj.attributes.hs_color ? Math.ceil(this.stateObj.attributes.hs_color[1]): 0;
|
||||
default:
|
||||
return 0;
|
||||
}
|
||||
}
|
||||
|
||||
get min() {
|
||||
switch (this.attribute) {
|
||||
case "color_temp":
|
||||
return this.stateObj.attributes.min_mireds;
|
||||
default:
|
||||
return 0;
|
||||
}
|
||||
}
|
||||
get max() {
|
||||
switch (this.attribute) {
|
||||
case "color_temp":
|
||||
return this.stateObj.attributes.max_mireds;
|
||||
case "red":
|
||||
case "green":
|
||||
case "blue":
|
||||
return 255;
|
||||
case "hue":
|
||||
return 360;
|
||||
default:
|
||||
return 100;
|
||||
}
|
||||
}
|
||||
|
||||
set _value(value) {
|
||||
let attr = this.attribute;
|
||||
let on = true;
|
||||
let _value;
|
||||
switch (attr) {
|
||||
case "brightness":
|
||||
value = Math.ceil(value/100.0*255);
|
||||
if (value) {
|
||||
if (!value) on = false;
|
||||
break;
|
||||
case "red":
|
||||
case "green":
|
||||
case "blue":
|
||||
_value = this.stateObj.attributes.rgb_color || [0,0,0];
|
||||
if (attr === "red") _value[0] = value;
|
||||
if (attr === "green") _value[1] = value;
|
||||
if (attr === "blue") _value[2] = value;
|
||||
value = _value;
|
||||
attr = "rgb_color";
|
||||
break;
|
||||
case "hue":
|
||||
case "saturation":
|
||||
_value = this.stateObj.attributes.hs_color || [0,0];
|
||||
if (attr === "hue") _value[0] = value;
|
||||
if (attr === "saturation") _value[1] = value;
|
||||
value = _value;
|
||||
attr = "hs_color";
|
||||
break;
|
||||
}
|
||||
|
||||
if (on) {
|
||||
this._hass.callService("light", "turn_on", {
|
||||
entity_id: this.stateObj.entity_id,
|
||||
brightness: value,
|
||||
[attr]: value,
|
||||
});
|
||||
} else {
|
||||
this._hass.callService("light", "turn_off", {
|
||||
@ -25,13 +95,42 @@ export class LightController extends Controller {
|
||||
get string() {
|
||||
if (this.stateObj.state === "off")
|
||||
return this._hass.localize("state.default.off");
|
||||
switch (this.attribute) {
|
||||
case "color_temp":
|
||||
return `${this.value} K`;
|
||||
case "brightness":
|
||||
case "saturation":
|
||||
return `${this.value} %`;
|
||||
case "hue":
|
||||
return `${this.value} °`;
|
||||
default:
|
||||
return this.value;
|
||||
}
|
||||
}
|
||||
|
||||
get hasSlider() {
|
||||
switch (this.attribute) {
|
||||
case "brightness":
|
||||
if ("brightness" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 1)) return true;
|
||||
case "color_temp":
|
||||
if ("color_temp" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 2)) return true;
|
||||
case "red":
|
||||
case "green":
|
||||
case "blue":
|
||||
if ("rgb_color" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 16)) return true;
|
||||
case "hue":
|
||||
case "saturation":
|
||||
if ("hs_color" in this.stateObj.attributes) return true;
|
||||
if (("supported_features" in this.stateObj.attributes) &&
|
||||
(this.stateObj.attributes.supported_features & 16)) return true;
|
||||
default:
|
||||
return false;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
Loading…
x
Reference in New Issue
Block a user