BREAKING CHANGES!! - Allow switching cards based on browser ID.
This commit is contained in:
parent
2b02f22084
commit
c9b8a16bb3
65
README.md
65
README.md
@ -1,11 +1,33 @@
|
|||||||
state-switch
|
state-switch
|
||||||
============
|
============
|
||||||
|
|
||||||
|
Allows you to display different cards depending on the state of an entity, the currently logged in user or the current device-browser combination
|
||||||
|
|
||||||
|
This card requires [card-tools](https://github.com/thomasloven/lovelace-card-tools) to be installed.
|
||||||
|
|
||||||
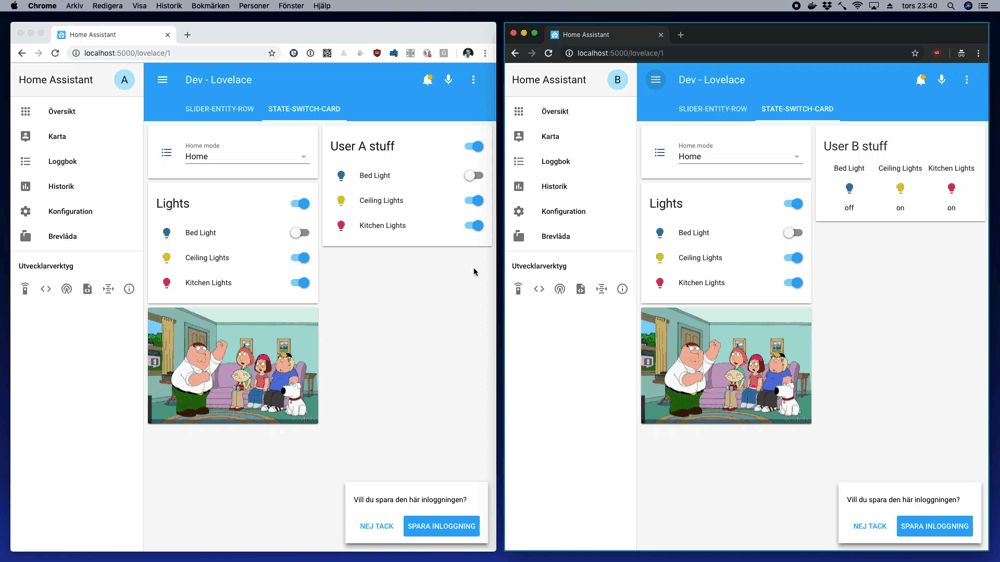
|
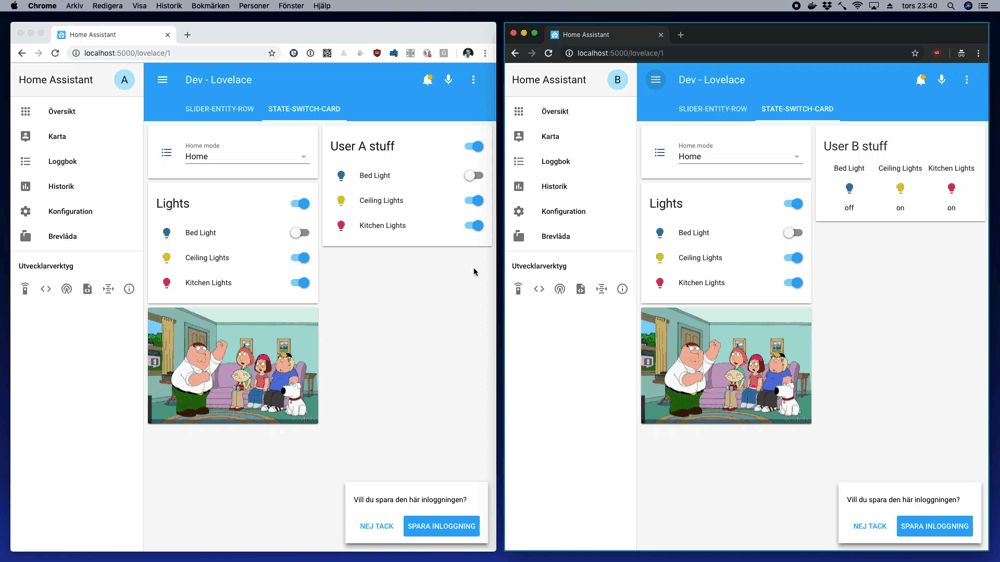
|
||||||
|
|
||||||
|
> Note in the animation above that the two browser windows have two different users logged in, which changes the rightmost card.
|
||||||
|
|
||||||
|
## Installation
|
||||||
|
|
||||||
|
Please read and follow this guide: [Lovelace Plugins](https://github.com/thomasloven/hass-config/wiki/Lovelace-Plugins)
|
||||||
|
|
||||||
|
## Options
|
||||||
|
|
||||||
|
| Name | Type | Default | Description
|
||||||
|
| ---- | ---- | ------- | -----------
|
||||||
|
| type | string | **Required** | `custom:state-switch`
|
||||||
|
| entity | string | **Required** | Controlling entity id, `user` or `browser`
|
||||||
|
| states | object | **Required** | Map of states to cards to display
|
||||||
|
| default | string | none | State to use as default
|
||||||
|
|
||||||
|
The `entity` parameter can take three different types of value
|
||||||
|
|
||||||
|
### Entity\_id
|
||||||
|
If the `entity` parameter is set to an entity id, which card is displayed will depend on the state of that entity.
|
||||||
|
|
||||||
```yaml
|
```yaml
|
||||||
- title: state-switch-card
|
|
||||||
cards:
|
cards:
|
||||||
- type: entities
|
- type: entities
|
||||||
entities:
|
entities:
|
||||||
@ -35,7 +57,27 @@ state-switch
|
|||||||
- light.bed_light
|
- light.bed_light
|
||||||
- light.ceiling_lights
|
- light.ceiling_lights
|
||||||
- light.kitchen_lights
|
- light.kitchen_lights
|
||||||
|
```
|
||||||
|
|
||||||
|
Note that the words `on` and `off` are magic in yaml, so if the entity is e.g. a switch, you need to quote the keys in the `states:` mapping:
|
||||||
|
|
||||||
|
```yaml
|
||||||
|
states:
|
||||||
|
"on":
|
||||||
|
type: markdown
|
||||||
|
content:>
|
||||||
|
Light is on
|
||||||
|
"off":
|
||||||
|
type: markdown
|
||||||
|
content:>
|
||||||
|
Light is off
|
||||||
|
```
|
||||||
|
|
||||||
|
### user
|
||||||
|
If the `entity` parameter is set to `user`, which card is displayed will depend on the currently logged in users username.
|
||||||
|
|
||||||
|
```yaml
|
||||||
|
cards:
|
||||||
- type: custom:state-switch
|
- type: custom:state-switch
|
||||||
entity: user
|
entity: user
|
||||||
default: default
|
default: default
|
||||||
@ -59,3 +101,24 @@ state-switch
|
|||||||
content: >
|
content: >
|
||||||
## Unknown user
|
## Unknown user
|
||||||
```
|
```
|
||||||
|
|
||||||
|
### browser
|
||||||
|
If the `entity` parameter is set to `browser`, which card is displayed will depend on the device-browser combination which is currently displaying the page.
|
||||||
|
|
||||||
|
This "browser ID" is in the form of a 16 character string with a dash in the middle and is unique, random and persistent for the browser.
|
||||||
|
|
||||||
|
If the `default` parameter is **not** set, the default behavior will be to display a small card containing the browser ID.
|
||||||
|
|
||||||
|
```yaml
|
||||||
|
- type: custom:state-switch
|
||||||
|
entity: browser
|
||||||
|
states:
|
||||||
|
'9c2aaf6f-ed26e3c1':
|
||||||
|
type: markdown
|
||||||
|
content: >
|
||||||
|
Desktop
|
||||||
|
'c8a4981c-d69c5e3c':
|
||||||
|
type: markdown
|
||||||
|
content: >
|
||||||
|
Mobile
|
||||||
|
```
|
||||||
|
@ -1,11 +1,9 @@
|
|||||||
class StateSwitch extends Polymer.Element{
|
var LitElement = LitElement || Object.getPrototypeOf(customElements.get('hui-error-entity-row'));
|
||||||
|
class StateSwitch extends LitElement {
|
||||||
|
|
||||||
setConfig(config) {
|
setConfig(config) {
|
||||||
this.config = config;
|
this.config = config;
|
||||||
|
|
||||||
this.root = document.createElement('div');
|
|
||||||
this.appendChild(this.root);
|
|
||||||
|
|
||||||
this.cardSize = 1;
|
this.cardSize = 1;
|
||||||
this.cards = {}
|
this.cards = {}
|
||||||
|
|
||||||
@ -19,6 +17,15 @@ class StateSwitch extends Polymer.Element{
|
|||||||
|
|
||||||
this.cardSize = Math.max(this.cardSize, card.getCardSize());
|
this.cardSize = Math.max(this.cardSize, card.getCardSize());
|
||||||
}
|
}
|
||||||
|
|
||||||
|
this.idCard = document.createElement("ha-card");
|
||||||
|
this.idCard.innerHTML = `<h2>${window.cardTools.deviceID}</h2>`;
|
||||||
|
}
|
||||||
|
|
||||||
|
render() {
|
||||||
|
return window.cardTools.litHtml`
|
||||||
|
<div id="root">${this.currentCard}</div>
|
||||||
|
`;
|
||||||
}
|
}
|
||||||
|
|
||||||
set hass(hass) {
|
set hass(hass) {
|
||||||
@ -27,15 +34,14 @@ class StateSwitch extends Polymer.Element{
|
|||||||
const lastCard = this.currentCard;
|
const lastCard = this.currentCard;
|
||||||
if (this.config.entity === 'user') {
|
if (this.config.entity === 'user') {
|
||||||
this.currentCard = this.cards[hass.user.name] || this.cards[this.config.default];
|
this.currentCard = this.cards[hass.user.name] || this.cards[this.config.default];
|
||||||
|
} else if(this.config.entity == 'browser') {
|
||||||
|
this.currentCard = this.cards[window.cardTools.deviceID] || (this.config.default)?this.cards[this.config.default]:this.idCard;
|
||||||
} else {
|
} else {
|
||||||
let state = hass.states[this.config.entity];
|
let state = hass.states[this.config.entity];
|
||||||
this.currentCard = ((state)?this.cards[state.state]:null) || this.cards[this.config.default];
|
this.currentCard = ((state)?this.cards[state.state]:null) || this.cards[this.config.default];
|
||||||
}
|
}
|
||||||
|
|
||||||
if(this.currentCard != lastCard) {
|
if(this.currentCard != lastCard) this.requestUpdate();
|
||||||
while(this.root.firstChild) this.root.removeChild(this.root.firstChild);
|
|
||||||
this.root.appendChild(this.currentCard);
|
|
||||||
}
|
|
||||||
|
|
||||||
for(var k in this.cards)
|
for(var k in this.cards)
|
||||||
this.cards[k].hass = hass;
|
this.cards[k].hass = hass;
|
||||||
|
Loading…
x
Reference in New Issue
Block a user