Add support for fan and input_select (#27)
Fix #18, fix #28 * Add support for fan domain * Fix fan status string when speeds are not numeric * Add support for input_select domain * Fan and input_select domains will change the max, min, and scale if all of their options (or speeds) are numeric * Perform numeric check less often * Remove numeric mode in favor of cleaner logic * Change some one-liners to arrow functions * Fix bug on getter for string based option lists * Update README.md
This commit is contained in:
parent
be80ffd6bc
commit
96ed393e5c
@ -1,7 +1,7 @@
|
||||
slider-entity-row
|
||||
=================
|
||||
|
||||
Add a slider to adjust brightness of lights, volume of media players or position of covers in lovelace entity cards
|
||||
Add a slider to adjust brightness of lights, volume of media players, position of covers and speed of fans in lovelace entity cards
|
||||
|
||||
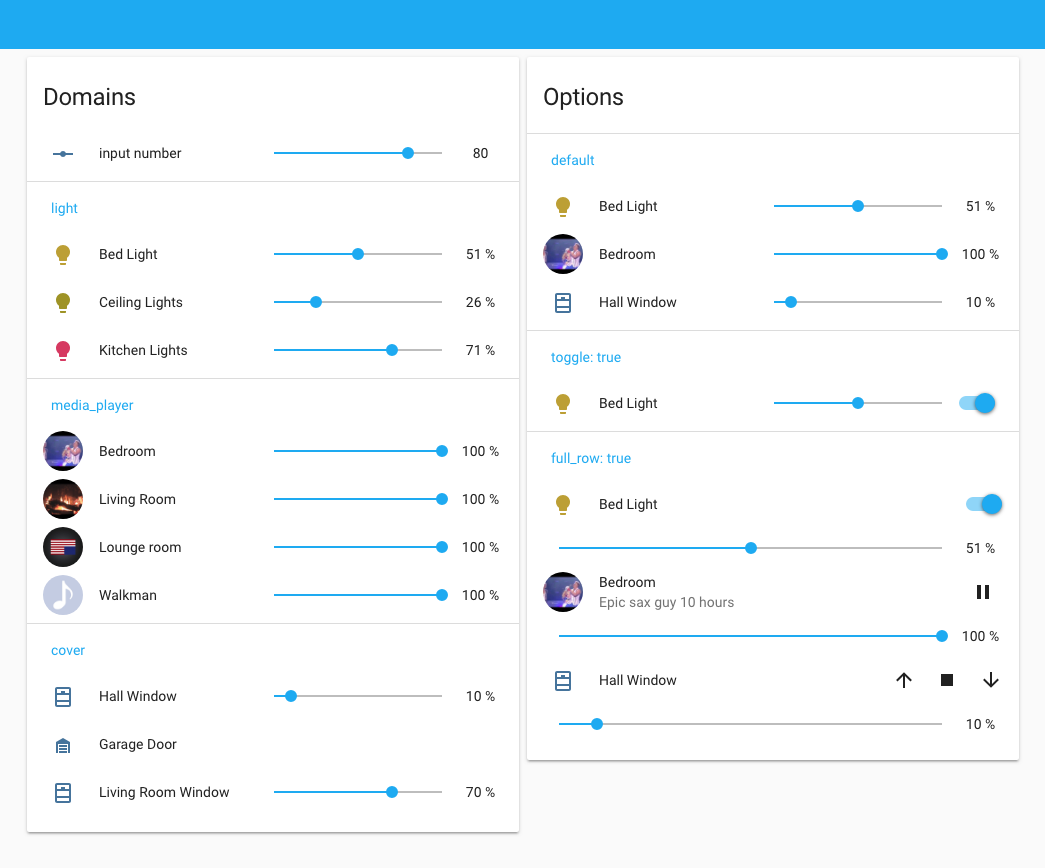
|
||||
|
||||
@ -86,3 +86,6 @@ Add a slider to adjust brightness of lights, volume of media players or position
|
||||
`step` - (default: 5) Step size of slider
|
||||
Note that slider values are in percent and will be rescaled e.g. for lights which require a brightness setting between 0 and 255.
|
||||
|
||||
|
||||
---
|
||||
Thanks to Gabe Cook (@gabe565) for help with fan support.
|
||||
|
@ -84,20 +84,24 @@ class SliderEntityRow extends Polymer.Element {
|
||||
else
|
||||
this._hass.callService('light', 'turn_off', { entity_id: stateObj.entity_id });
|
||||
},
|
||||
get: (stateObj) => {
|
||||
return (stateObj.state === 'on')?Math.ceil(stateObj.attributes.brightness*100.0/255):0;
|
||||
},
|
||||
supported: (stateObj) => {
|
||||
if(stateObj.state === 'off' && this._config.hide_when_off) return false;
|
||||
if('brightness' in stateObj.attributes) return true;
|
||||
if(('supported_features' in stateObj.attributes) &&
|
||||
(stateObj.attributes.supported_features & 1)) return true;
|
||||
return false;
|
||||
get: (stateObj) => (stateObj.state === 'on')?Math.ceil(stateObj.attributes.brightness*100.0/255):0,
|
||||
supported: {
|
||||
slider: (stateObj) => {
|
||||
if(stateObj.state === 'off' && this._config.hide_when_off) return false;
|
||||
if('brightness' in stateObj.attributes) return true;
|
||||
if(('supported_features' in stateObj.attributes) &&
|
||||
(stateObj.attributes.supported_features & 1)) return true;
|
||||
return false;
|
||||
},
|
||||
toggle: () => true,
|
||||
},
|
||||
string: (stateObj, l18n) => {
|
||||
if(stateObj.state === 'off') return l18n['state.default.off'];
|
||||
return `${this.controller.get(stateObj)} %`;
|
||||
},
|
||||
min: () => 0,
|
||||
max: () => 100,
|
||||
step: () => 5,
|
||||
},
|
||||
|
||||
media_player: {
|
||||
@ -105,16 +109,18 @@ class SliderEntityRow extends Polymer.Element {
|
||||
value = value/100.0;
|
||||
this._hass.callService('media_player', 'volume_set', { entity_id: stateObj.entity_id, volume_level: value });
|
||||
},
|
||||
get: (stateObj) => {
|
||||
return (stateObj.attributes.is_volume_muted)?0:Math.ceil(stateObj.attributes.volume_level*100.0);
|
||||
},
|
||||
supported: (stateObj) => {
|
||||
return true;
|
||||
get: (stateObj) => (stateObj.attributes.is_volume_muted)?0:Math.ceil(stateObj.attributes.volume_level*100.0),
|
||||
supported: {
|
||||
slider: () => true,
|
||||
toggle: () => false,
|
||||
},
|
||||
string: (stateObj, l18n) => {
|
||||
if (stateObj.attributes.is_volume_muted) return '-';
|
||||
return `${this.controller.get(stateObj)} %`;
|
||||
},
|
||||
min: () => 0,
|
||||
max: () => 100,
|
||||
step: () => 5,
|
||||
},
|
||||
|
||||
cover: {
|
||||
@ -124,20 +130,62 @@ class SliderEntityRow extends Polymer.Element {
|
||||
else
|
||||
this._hass.callService('cover', 'close_cover', { entity_id: stateObj.entity_id });
|
||||
},
|
||||
get: (stateObj) => {
|
||||
return (stateObj.state === 'open')?Math.ceil(stateObj.attributes.current_position):0;
|
||||
},
|
||||
supported: (stateObj) => {
|
||||
if('current_position' in stateObj.attributes) return true;
|
||||
if(('supported_features' in stateObj.attributes) &&
|
||||
(stateObj.attributes.supported_features & 4)) return true;
|
||||
return false;
|
||||
get: (stateObj) => (stateObj.state === 'open')?Math.ceil(stateObj.attributes.current_position):0,
|
||||
supported: {
|
||||
slider: (stateObj) => {
|
||||
if(stateObj.attributes.hasOwnProperty('current_position')) return true;
|
||||
if((stateObj.attributes.hasOwnProperty('supported_features')) &&
|
||||
(stateObj.attributes.supported_features & 4)) return true;
|
||||
return false;
|
||||
},
|
||||
toggle: () => false,
|
||||
},
|
||||
string: (stateObj, l18n) => {
|
||||
if (!this.controller.supported(stateObj)) return '';
|
||||
if (stateObj.state === 'closed') return l18n['state.cover.closed'];
|
||||
return `${this.controller.get(stateObj)} %`;
|
||||
},
|
||||
min: () => 0,
|
||||
max: () => 100,
|
||||
step: () => 5,
|
||||
},
|
||||
|
||||
fan: {
|
||||
set: (stateObj, value) => {
|
||||
--value;
|
||||
if (value in stateObj.attributes.speed_list)
|
||||
this._hass.callService('fan', 'turn_on', { entity_id: stateObj.entity_id, speed: stateObj.attributes.speed_list[value] });
|
||||
else
|
||||
this._hass.callService('fan', 'turn_off', { entity_id: stateObj.entity_id });
|
||||
},
|
||||
get: (stateObj) => (stateObj.state !== 'off')?stateObj.attributes.speed_list.indexOf(stateObj.attributes.speed)+1:0,
|
||||
supported: {
|
||||
slider: (stateObj) => !(stateObj.state === 'off' && this._config.hide_when_off) && stateObj.attributes.hasOwnProperty('speed'),
|
||||
toggle: () => true,
|
||||
},
|
||||
string: (stateObj, l18n) => {
|
||||
if(stateObj.state === 'off') return l18n['state.default.off'];
|
||||
return stateObj.attributes.speed;
|
||||
},
|
||||
min: (stateObj) => 0,
|
||||
max: (stateObj) => stateObj.attributes.speed_list.length,
|
||||
step: () => 1,
|
||||
},
|
||||
|
||||
input_select: {
|
||||
set: (stateObj, value) => {
|
||||
if (value in stateObj.attributes.options)
|
||||
this._hass.callService('input_select', 'select_option', { entity_id: stateObj.entity_id, option: stateObj.attributes.options[value] });
|
||||
},
|
||||
get: (stateObj) => stateObj.attributes.options.indexOf(stateObj.state),
|
||||
supported: {
|
||||
slider: (stateObj) => stateObj.attributes.hasOwnProperty('options') && stateObj.attributes.options.length > 1,
|
||||
toggle: () => false,
|
||||
},
|
||||
string: (stateObj) => stateObj.state,
|
||||
min: () => 0,
|
||||
max: (stateObj) => stateObj.attributes.options.length-1,
|
||||
step: () => 1,
|
||||
},
|
||||
};
|
||||
|
||||
@ -149,7 +197,7 @@ class SliderEntityRow extends Polymer.Element {
|
||||
if(!this.controller) throw new Error('Unsupported entity domain: ' + domain);
|
||||
|
||||
this.displayRow = !config.full_row;
|
||||
this.displayToggle = config.toggle && domain === 'light';
|
||||
this.displayToggle = config.toggle && this.controller.supported.toggle();
|
||||
this.displayValue = !this.displayToggle;
|
||||
if(config.hide_state) this.displayValue = false;
|
||||
this.displaySlider = false;
|
||||
@ -161,8 +209,11 @@ class SliderEntityRow extends Polymer.Element {
|
||||
if(this._hass && this._config) {
|
||||
this.stateObj = this._config.entity in this._hass.states ? this._hass.states[this._config.entity] : null;
|
||||
if(this.stateObj) {
|
||||
this.min = this._config.min || this.controller.min(this.stateObj);
|
||||
this.max = this._config.max || this.controller.max(this.stateObj);
|
||||
this.step = this._config.step || this.controller.step(this.stateObj);
|
||||
this.value = this.controller.get(this.stateObj);
|
||||
this.displaySlider = this.controller.supported(this.stateObj);
|
||||
this.displaySlider = this.controller.supported.slider(this.stateObj);
|
||||
}
|
||||
}
|
||||
}
|
||||
@ -179,8 +230,11 @@ class SliderEntityRow extends Polymer.Element {
|
||||
if(hass && this._config) {
|
||||
this.stateObj = this._config.entity in hass.states ? hass.states[this._config.entity] : null;
|
||||
if(this.stateObj) {
|
||||
this.min = this._config.min || this.controller.min(this.stateObj);
|
||||
this.max = this._config.max || this.controller.max(this.stateObj);
|
||||
this.step = this._config.step || this.controller.step(this.stateObj);
|
||||
this.value = this.controller.get(this.stateObj);
|
||||
this.displaySlider = this.controller.supported(this.stateObj);
|
||||
this.displaySlider = this.controller.supported.slider(this.stateObj);
|
||||
}
|
||||
}
|
||||
}
|
||||
|
Loading…
x
Reference in New Issue
Block a user